Disclaimer: There may be newer/simpler ways of doing this now that SearchKit has a "autocomplete" display, but I have not experimented with it yet, and not sure if we can use it to override a EntityReference field.
In a custom extension, you can implement an auto-complete provider that overrides the default behaviour. Here is an example.
- add the
[email protected]
mixin to the info.xml
file of your extension:
<mixins>
[...]
<mixin>[email protected]</mixin>
</mixins>
Then create the directory for the service, where we will create the PHP file:
mkdir -p Civi/Api4/Service/Autocomplete/
Now create a file called Civi/Api4/Service/Autocomplete/MyAddressAutocompleteProvider.php
with the following contents:
<?php
namespace Civi\Api4\Service\Autocomplete;
use Civi;
use Civi\Core\Event\GenericHookEvent;
use Civi\Core\HookInterface;
/**
* @service
* @internal
*/
class MyAddressAutocompleteProvider extends \Civi\Core\Service\AutoService implements HookInterface {
/**
* Provide default SearchDisplay for Address autocompletes
*
* @param \Civi\Core\Event\GenericHookEvent $e
*/
public function on_civi_search_defaultDisplay(GenericHookEvent $event): void {
if ($event->display['type'] !== 'autocomplete' || $event->savedSearch['api_entity'] !== 'Address') {
return;
}
$event->display['settings'] = [
'sort' => [
['street_address', 'ASC'],
],
'columns' => [
[
'type' => 'field',
'key' => 'street_address',
'rewrite' => '[street_address], [city]',
'empty_value' => '[city]',
],
[
'type' => 'field',
'key' => 'state_province_id.name',
'rewrite' => '[postal_code], [state_province_id.name], [country_id.name]',
'empty_value' => '[country_id.name]',
],
[
'type' => 'field',
'key' => 'id',
'rewrite' => '#[id]',
],
],
];
}
}
You can rename MyAddressAutocompleteProvider
to something more relevant to your extension name, but avoid calling it just AddressAutocompleteProvider
because that's what it is called in CiviCRM core.
Notice in my example above, that I added the [postal_code]
as part of the second line, before the state/province. When fields are added in the rewrite, they are automatically added to the SQL query for autocompletes.
For all of this to work, you will need to flush the CiviCRM cache (either from the admin menu, or cv flush
on the command line).
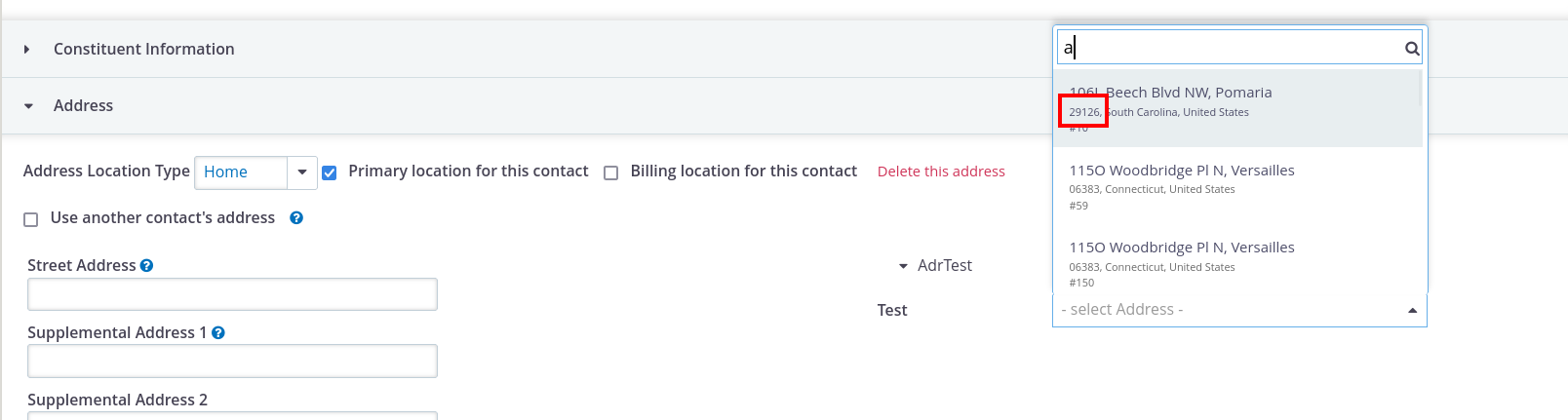
This example was based on the official documentation, although some bits were not obvious to me at first.